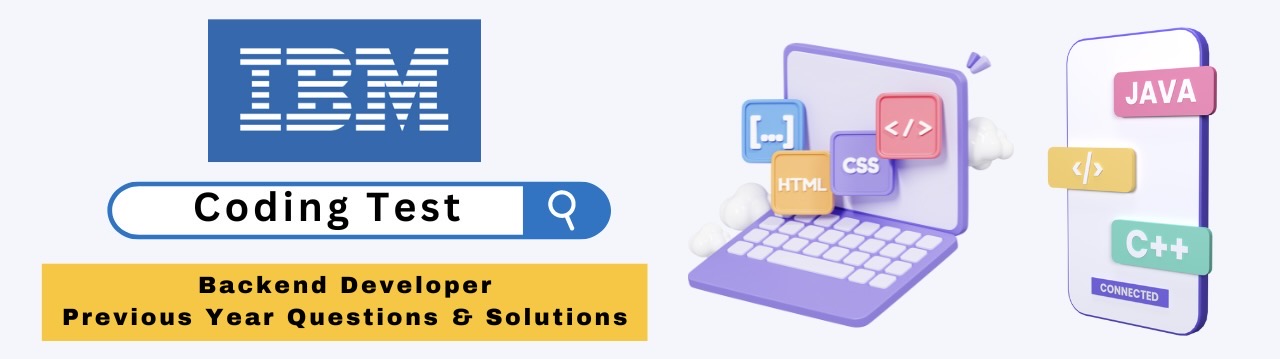
IBM Coding Exam 2024 | Problem & Solutions | Software Developer Role
In This Post We Are Solving Questions Which Appeared In IBM Coding Exam 2024 For The Software Developer Role, Total 3 Problems Solved In This Post.
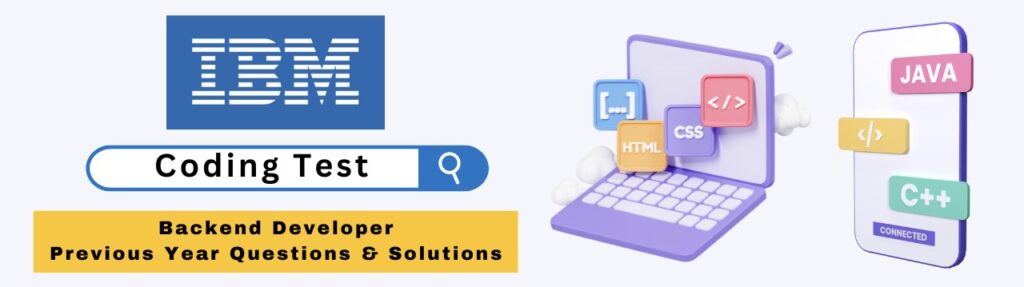
Problem Statement – IBM Coding Exam 2024
You need to validate email addresses based on specific rules. A valid email address follows this pattern:
- The user part of the email must start with 1 to 6 lowercase English letters
[a-z]
. - It may optionally be followed by an underscore
_
. - After the optional underscore, it may have up to 4 digits
[0-9]
. - The domain part must be exactly
hackerrank.com
.
Given n
email addresses, determine if each email address is valid or not.
Input Format – IBM Coding Exam 2024
- The first line contains an integer
n
, the number of email addresses. - Each of the next
n
lines contains one email address to be validated.
Output Format
- For each email address, print
True
if it is valid, otherwise printFalse
.
Example
Input: IBM Coding Exam 2024
5
julia@hackerrank.com
julia_@hackerrank.com
julia_0@hackerrank.com
julia0_@hackerrank.com
julia@gmail.com
Output: IBM Coding Exam 2024
True
True
True
False
False
Explanation
julia@hackerrank.com
– Valid: Starts with lowercase letters and has a valid domain.julia_@hackerrank.com
– Valid: Starts with lowercase letters, has an underscore, and a valid domain.julia_0@hackerrank.com
– Valid: Starts with lowercase letters, has an underscore, a digit, and a valid domain.julia0_@hackerrank.com
– Invalid: Digits cannot precede the underscore.julia@gmail.com
– Invalid: Domain is nothackerrank.com
.
Solution – IBM Coding Exam 2024
Java Solution
import java.util.Scanner;
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class Solution {
final static Scanner scan = new Scanner(System.in);
final static String regularExpression = "^[a-z]{1,6}_?[0-9]{0,4}@hackerrank\\.com$";
public static void main(String[] args) {
int query = Integer.parseInt(scan.nextLine());
String[] result = new String[query];
for (int i = 0; i < query; i++) {
String someString = scan.nextLine();
if (someString.matches(regularExpression)) {
result[i] = "True";
} else {
result[i] = "False";
}
}
for (String res : result) {
System.out.println(res);
}
}
}
Python Solution
import re
import sys
# Regular expression for valid HackerRank emails
regular_expression = r'^[a-z]{1,6}_?[0-9]{0,4}@hackerrank\.com$'
def main():
input = sys.stdin.read().splitlines()
query = int(input[0])
result = ["False"] * query
for i in range(1, query + 1):
some_string = input[i]
if re.match(regular_expression, some_string):
result[i - 1] = "True"
for res in result:
print(res)
if __name__ == "__main__":
main()
Explanation
Regular Expression: IBM Coding Exam 2024
^[a-z]{1,6}_?[0-9]{0,4}@hackerrank\.com$
^[a-z]{1,6}
: Start with 1 to 6 lowercase letters._?
: Followed by an optional underscore.[0-9]{0,4}
: Followed by up to 4 digits.@hackerrank\.com$
: Ending with@hackerrank.com
.
Java Implementation:
- Read the number of queries.
- Initialize a
result
array to store the validation results. - Iterate over the input emails, validate each using
matches()
method with the regex, and store the result. - Print each result.
Python Implementation:
- Read all input lines using
sys.stdin.read().splitlines()
. - Extract the number of queries.
- Initialize a
result
list with “False” values. - Iterate over the input emails, validate each using
re.match()
with the regex, and store the result. - Print each result.
Both solutions follow the same logic but are implemented in different languages. The Java version uses matches()
method from String
class, while the Python version uses re.match()
from the re
module.
Problem Breakdown
Input:
input1
: An integer representing the number of files, N.input2
: A list of strings representing the file names.
Output:
- A string containing the first letters of the file names sorted in alphabetical order.
- If there are no files (
input1
is 0), return “0”.
Steps:
- Extract the first letter from each file name.
- Sort the extracted letters.
- Join the sorted letters into a string and return it.
Python Solution – IBM Coding Exam 2024
class UserMainCode(object):
@classmethod
def uniqueFiles(cls, input1, input2):
if input1 == 0:
return "0"
first_letters = [file[0] for file in input2]
sorted_letters = sorted(first_letters)
return "".join(sorted_letters)
# Example usage
input1 = 4
input2 = ["name", "editsurname", "edit", "editing"]
result = UserMainCode.uniqueFiles(input1, input2)
print(result) # Output: eeen
Java Solution
import java.util.Arrays;
public class UserMainCode {
public static String uniqueFiles(int input1, String[] input2) {
if (input1 == 0) {
return "0";
}
char[] firstLetters = new char[input1];
for (int i = 0; i < input1; i++) {
firstLetters[i] = input2[i].charAt(0);
}
Arrays.sort(firstLetters);
return new String(firstLetters);
}
public static void main(String[] args) {
int input1 = 4;
String[] input2 = {"name", "editsurname", "edit", "editing"};
System.out.println(uniqueFiles(input1, input2)); // Output: eeen
}
}
C Solution – IBM Coding Exam 2024
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char* uniqueFiles(int input1, char** input2) {
if (input1 == 0) {
return "0";
}
char* firstLetters = (char*)malloc(input1 * sizeof(char));
for (int i = 0; i < input1; i++) {
firstLetters[i] = input2[i][0];
}
qsort(firstLetters, input1, sizeof(char), (int(*)(const void*, const void*))strcmp);
return firstLetters;
}
int main() {
int input1 = 4;
char* input2[] = {"name", "editsurname", "edit", "editing"};
char* result = uniqueFiles(input1, input2);
printf("%s\n", result); // Output: eeen
free(result);
return 0;
}
C++ Solution – IBM Coding Exam 2024
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class UserMainCode {
public:
static string uniqueFiles(int input1, vector<string>& input2) {
if (input1 == 0) {
return "0";
}
vector<char> firstLetters(input1);
for (int i = 0; i < input1; i++) {
firstLetters[i] = input2[i][0];
}
sort(firstLetters.begin(), firstLetters.end());
return string(firstLetters.begin(), firstLetters.end());
}
};
int main() {
int input1 = 4;
vector<string> input2 = {"name", "editsurname", "edit", "editing"};
cout << UserMainCode::uniqueFiles(input1, input2) << endl; // Output: eeen
return 0;
}
Explanation – IBM Coding Exam 2024
Python:
- Use list comprehension to extract the first letters.
- Sort the letters using the built-in
sorted
function. - Join the sorted letters into a string.
Java:
- Use an array to store the first letters.
- Sort the array using
Arrays.sort
. - Convert the sorted array to a string.
C:
- Use dynamic memory allocation to store the first letters.
- Sort the array using
qsort
. - Return the sorted array as a string.
C++:
- Use a vector to store the first letters.
- Sort the vector using
std::sort
. - Convert the sorted vector to a string.
Each solution follows the same logical steps but uses language-specific features and libraries to achieve the result.
Problem Description – IBM Coding Exam 2024
A financial services company is uploading documents to a compliance system for analysis. Each document is divided into equal-sized packets. These packets are then grouped into “chunks” of packets for upload, where each chunk is a contiguous collection of packets. The company uploads chunks until the entire document is completely uploaded. Given a document that is partially uploaded, the goal is to determine the minimum number of chunks that are yet to be uploaded.
Input:
totalPackets
: An integer representing the total number of packets in the document.uploadedChunks
: A 2D array where each element represents a chunk already uploaded in the form of[start, end]
.
Output:
- An integer representing the minimum number of chunks that need to be uploaded to complete the document upload.
Example:
Input:
totalPackets = 10
uploadedChunks = [[1, 2], [9, 10]]
Output:
2
Explanation:
The document has 10 packets. The chunks [1, 2]
and [9, 10]
have already been uploaded. The remaining packets are [3, 4, 5, 6, 7, 8]
. These can be uploaded in two chunks: [3, 4, 5, 6]
and [7, 8]
.
Java Solution
import java.util.*;
public class Solution {
public static int minimumChunksRequired(long totalPackets, int[][] uploadedChunks) {
// Initialize an array to mark uploaded packets
boolean[] uploaded = new boolean[(int) totalPackets + 1];
// Mark the uploaded packets
for (int[] chunk : uploadedChunks) {
for (int i = chunk[0]; i <= chunk[1]; i++) {
uploaded[i] = true;
}
}
// Find gaps (ranges of missing packets)
List<int[]> gaps = new ArrayList<>();
int start = 0;
for (int i = 1; i <= totalPackets; i++) {
if (!uploaded[i]) {
if (start == 0) {
start = i;
}
} else {
if (start != 0) {
gaps.add(new int[]{start, i - 1});
start = 0;
}
}
}
if (start != 0) {
gaps.add(new int[]{start, (int) totalPackets});
}
// Calculate the minimum number of chunks to cover the gaps
int chunksNeeded = 0;
for (int[] gap : gaps) {
int gapSize = gap[1] - gap[0] + 1;
// Use chunks of size 4 first
chunksNeeded += gapSize / 4;
gapSize %= 4;
// Use chunks of size 2
chunksNeeded += gapSize / 2;
gapSize %= 2;
// Use chunks of size 1
chunksNeeded += gapSize;
}
return chunksNeeded;
}
public static void main(String[] args) {
// Sample Input
long totalPackets = 10;
int[][] uploadedChunks = { {1, 2}, {9, 10} };
// Function call
int result = minimumChunksRequired(totalPackets, uploadedChunks);
// Output the result
System.out.println(result); // Expected Output: 2
}
}
Python Solution – IBM Coding Exam 2024
def minimum_chunks_required(total_packets, uploaded_chunks):
# Initialize a list to mark uploaded packets
uploaded = [False] * (total_packets + 1)
# Mark the uploaded packets
for chunk in uploaded_chunks:
for i in range(chunk[0], chunk[1] + 1):
uploaded[i] = True
# Find gaps (ranges of missing packets)
gaps = []
start = 0
for i in range(1, total_packets + 1):
if not uploaded[i]:
if start == 0:
start = i
else:
if start != 0:
gaps.append((start, i - 1))
start = 0
if start != 0:
gaps.append((start, total_packets))
# Calculate the minimum number of chunks to cover the gaps
chunks_needed = 0
for gap in gaps:
gap_size = gap[1] - gap[0] + 1
# Use chunks of size 4 first
chunks_needed += gap_size // 4
gap_size %= 4
# Use chunks of size 2
chunks_needed += gap_size // 2
gap_size %= 2
# Use chunks of size 1
chunks_needed += gap_size
return chunks_needed
# Sample Input
total_packets = 10
uploaded_chunks = [(1, 2), (9, 10)]
# Function call
result = minimum_chunks_required(total_packets, uploaded_chunks)
# Output the result
print(result) # Expected Output: 2
Detailed Explanation – IBM Coding Exam 2024
Initialisation: IBM Coding Exam 2024
- Java: Initialise a
boolean
arrayuploaded
of sizetotalPackets + 1
to keep track of which packets have been uploaded. - Python: Initialise a list
uploaded
of sizetotal_packets + 1
to keep track of which packets have been uploaded.
Marking Uploaded Packets:
- Iterate through the
uploadedChunks
list and mark the corresponding indices in theuploaded
array/list astrue
(orFalse
).
Finding Gaps:
- Traverse the
uploaded
array/list to identify contiguous gaps where packets have not been uploaded. Each gap is represented as a start and end index.
Calculating Minimum Chunks: IBM Coding Exam 2024
- For each gap, calculate the minimum number of chunks required using a greedy approach:
- First, use chunks of size 4.
- Then, use chunks of size 2.
- Finally, use chunks of size 1.
This approach ensures that the gaps are covered using the fewest possible chunks, thereby minimising the number of chunks required to complete the upload.