Infosys Coding Exam Solutions – In This Post We Will Solve Coding Problems Statements From Infosys Specialist Programmer & Power Programmer Roles Which Includes Questions Asked in Previous Infosys Examination.
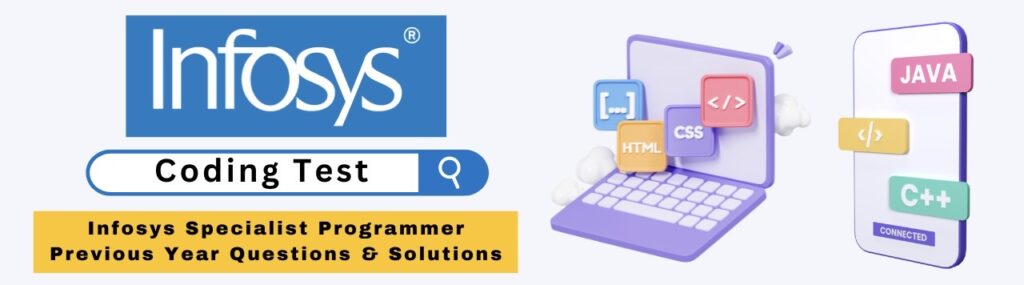
Question Number 1 – Infosys Coding Exam Solutions
You are developing a feature for a health and fitness app that helps users track their daily steps. Users input the total number of daily steps ( S ), the number of steps they have completed ( D ), and the number of days they have been tracking their steps ( N ). Your task is to find and return an integer value representing the average number of steps the user needs to take per day to reach their target.
Note: Round off the output to its nearest value.
Input Specification: Infosys Coding Exam Solutions
input1
: An integer value ( S ) representing the total number of daily steps.input2
: An integer value ( D ) representing the number of steps user has completed.input3
: An integer value ( N ) representing the number of days the user has been tracking their steps.
Output Specification: Infosys Coding Exam Solutions
- Return an integer value representing the average number of steps the user needs to take per day to reach their target.
Function Description:
class UserMainCode(object):
@classmethod
def goalTracker(cls, input1, input2, input3):
# write your code here
Example
Example 1: Infosys Coding Exam Solutions
- Input:
input1: 348
input2: 327
input3: 5
- Output:
4
- Explanation:
Here, ( S = 348 ), ( D = 327 ), and ( N = 5 ). The user needs to take 21 more steps (348 – 327 = 21). Since they have been tracking their steps for 5 days, the average steps per day needed to reach the target is ( \frac{21}{5} ), which is approximately 4. Hence, 4 is returned as output.
Answer
The output for the given example is 4
.
Solution with Explanation – Infosys Coding Exam Solutions
To solve this problem, follow these steps:
- Calculate Remaining Steps: Determine the number of steps the user still needs to take by subtracting the completed steps ( D ) from the total steps ( S ).
- Calculate Average Steps Per Day Needed: Divide the remaining steps by the number of days ( N ) and round the result to the nearest integer.
Here’s the implementation of the solution:
class UserMainCode:
@classmethod
def goalTracker(cls, S, D, N):
# Calculate remaining steps needed
remaining_steps = S - D
# Calculate average steps per day needed (round to nearest integer)
average_steps_per_day = round(remaining_steps / N)
return average_steps_per_day
Explanation: Infosys Coding Exam Solutions
- Remaining Steps Calculation:
remaining_steps = S - D
- This calculates the steps left to reach the goal. For example, if ( S = 348 ) and ( D = 327 ), then
remaining_steps
is ( 348 – 327 = 21 ).
- Average Steps Per Day Calculation:
average_steps_per_day = round(remaining_steps / N)
- This calculates the average steps needed per day by dividing the remaining steps by the number of days ( N ) and rounding to the nearest integer. For example, if
remaining_steps
is 21 and ( N = 5 ), thenaverage_steps_per_day
is ( \text{round}(21 / 5) = 4 ).
- Return the Result: Infosys Coding Exam Solutions
return average_steps_per_day
By following these steps, the function correctly calculates the average number of steps the user needs to take per day to reach their target.
Question Number 2
Problem Statement – Infosys Coding Exam Solutions
John is given a binary array of N
integers, where each integer is either 0
or 1
. He needs to convert all the integers in the array to either 0
or 1
. The cost of converting a 0
to 1
is X
coins and the cost of converting a 1
to 0
is Y
coins. Your task is to find and return the minimum amount of coins needed to convert the entire array to the same value (either all 0
s or all 1
s).
Input Specification:
input1
: An integerN
, representing the length of the binary array.input2
: An integerX
, representing the cost of converting0
to1
.input3
: An integerY
, representing the cost of converting1
to0
.input4
: An integer array of lengthN
, representing the binary array.
Output Specification:
- Return an integer value representing the minimum amount of coins needed to convert the array as desired.
Example:
Input:
input1: 4
input2: 5
input3: 4
input4: [1, 1, 1, 0]
Output:
5
Explanation:
Here, the given array is [1, 1, 1, 0]
.
- The cost of converting the array to
[1, 1, 1, 1]
is5
(converting the last0
to1
). - The cost of converting the array to
[0, 0, 0, 0]
is12
(converting each1
to0
).
Therefore, the optimal solution is to convert the last 0
to 1
, resulting in a cost of 5
.
Solution Explanation – Infosys Coding Exam Solutions
To solve this problem, we need to consider two potential conversion strategies:
- Converting the entire array to all
0
s. - Converting the entire array to all
1
s.
We can calculate the cost for each strategy and then choose the one with the minimum cost:
- To convert the array to all
0
s, sum the cost of converting every1
to0
. - To convert the array to all
1
s, sum the cost of converting every0
to1
.
Here’s the step-by-step approach in Python:
- Initialize
cost_to_convert_all_to_0
to0
. This will keep track of the cost to convert the array to all0
s. - Initialize
cost_to_convert_all_to_1
to0
. This will keep track of the cost to convert the array to all1
s. - Iterate through the array:
- If the element is
0
, addX
tocost_to_convert_all_to_1
. - If the element is
1
, addY
tocost_to_convert_all_to_0
.
- The minimum cost will be the lesser of the two computed costs.
Python Code – Infosys Coding Exam Solutions
class UserMainCode:
@classmethod
def minimumcost(cls, N, X, Y, arr):
cost_to_convert_all_to_0 = 0
cost_to_convert_all_to_1 = 0
for num in arr:
if num == 0:
cost_to_convert_all_to_1 += X
elif num == 1:
cost_to_convert_all_to_0 += Y
return min(cost_to_convert_all_to_0, cost_to_convert_all_to_1)
# Example usage:
N = 4
X = 5
Y = 4
arr = [1, 1, 1, 0]
print(UserMainCode.minimumcost(N, X, Y, arr)) # Output: 5
Detailed Explanation – Infosys Coding Exam Solutions
- Initialization: Start by initializing two variables to hold the conversion costs for both scenarios.
- Iteration: Traverse through each element in the array. Depending on the value of the element (
0
or1
), add the respective conversion cost to the corresponding variable. - Comparison: Finally, compare the total costs of the two scenarios and return the minimum value.
By following these steps, we ensure that we calculate the minimal cost required to convert the entire array to either all 0
s or all 1
s.