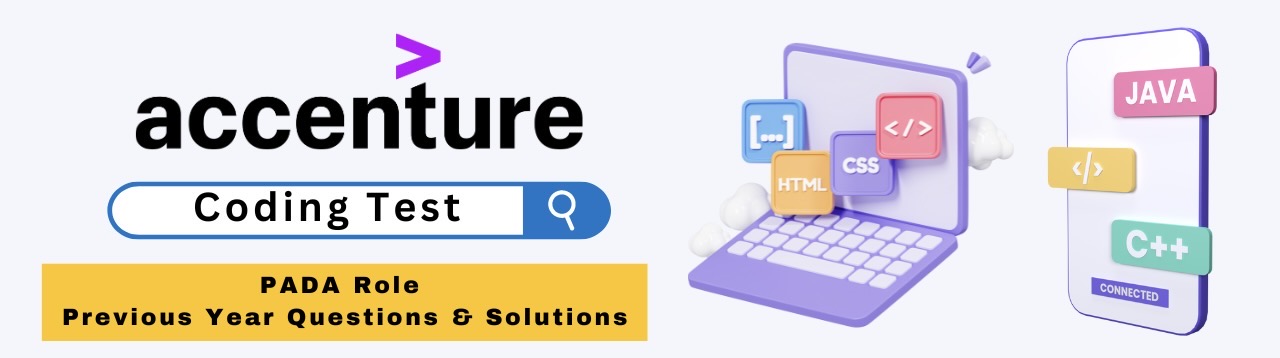
Accenture Coding Test | PADA Role 2024 | Questions & Answers
Accenture Coding Test – In This Article We Are Solving Previous Year Accenture Coding Test Questions With Solutions
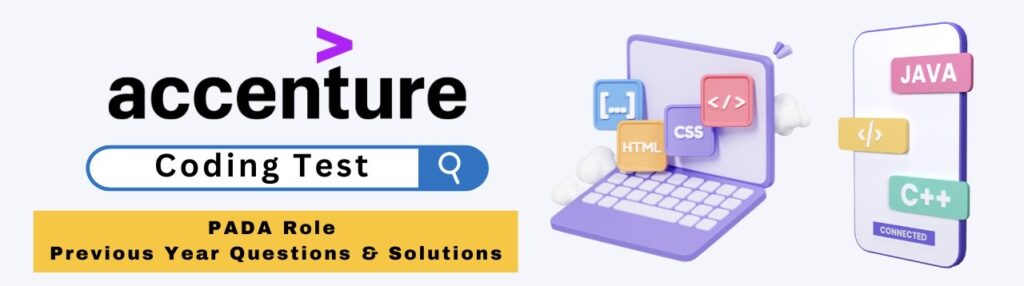
Problem Statement #1 – Accenture Coding Test
In this challenge, determine the minimum number of chairs to be purchased to accommodate all workers in a new business workroom. There is no chair at the beginning.
You will be given a string array of simulations. Each simulation is described by a combination of four characters: C, R, U, and L.
- C: A new employee arrives in the workroom. If there is a chair available, the employee takes it. Otherwise, a new one is purchased.
- R: An employee goes to a meeting room, freeing up a chair.
- U: An employee arrives from a meeting room. If there is a chair available, the employee takes it. Otherwise, a new one is purchased.
- L: An employee leaves the workroom, freeing up a chair.
Example – Accenture Coding Test
Given an array of strings representing the simulations:
a = ["CRUL"]
In this case, there is only one simulation, “CRUL”, represented in the table below:
Action | Total Chairs | Available Chairs |
---|---|---|
– | 0 | 0 |
C | 1 | 0 |
R | 1 | 1 |
U | 1 | 0 |
L | 1 | 1 |
- At first, there are 0 chairs.
- “C”: A new employee arrives in the workroom and one chair was purchased.
- “R”: An employee goes to the meeting room, freeing up a chair.
- “U”: An employee arrives from the meeting room, took the available chair.
- “L”: An employee leaves the workroom, freeing up a chair.
The minimum number of chairs to be purchased is one chair in this case. Thus, the result is [1]
.
Function Description – Accenture Coding Test
Complete the minChairs
function in the editor below.
minChairs
has the following parameter:
string simulations[n]
: an array of strings representing discrete simulations to process.
Returns – Accenture Coding Test
int[n]
: an array of integers denoting the minimal number of chairs required for each simulation.
Input Format
- The first line contains an integer,
n
, the size of the string array of simulations. - Each of the next
n
lines contains a string describingsimulations[i]
.
Sample Input
3
CCRUCL
CRUC
CCCC
Sample Output
3
2
4
Explanation – Accenture Coding Test
For the first simulation “CCRUCL”:
Action | Total Chairs | Available Chairs |
---|---|---|
– | 0 | 0 |
C | 1 | 0 |
C | 2 | 0 |
R | 2 | 1 |
U | 2 | 0 |
C | 3 | 0 |
L | 3 | 1 |
- “C”: A new employee arrives in the workroom and one chair was purchased.
- “C”: A new employee arrives in the workroom and the second chair was purchased.
- “R”: An employee goes to the meeting room, freeing up a chair.
- “U”: An employee arrives from the meeting room, took the available chair.
- “C”: A new employee arrives in the workroom and the third chair was purchased.
- “L”: An employee leaves the workroom, freeing up a chair.
The minimal number of chairs required after the simulation is 3.
For the second simulation “CRUC”:
Action | Total Chairs | Available Chairs |
---|---|---|
– | 0 | 0 |
C | 1 | 0 |
R | 1 | 1 |
U | 1 | 0 |
C | 2 | 0 |
The minimal number of chairs required after the simulation is 2.
For the third simulation “CCCC”:
Action | Total Chairs | Available Chairs |
---|---|---|
– | 0 | 0 |
C | 1 | 0 |
C | 2 | 0 |
C | 3 | 0 |
C | 4 | 0 |
The minimal number of chairs required after the simulation is 4.
Solution – Accenture Coding Test
Here is the Python function to solve the problem:
def minChairs(simulations):
results = []
for simulation in simulations:
total_chairs = 0
available_chairs = 0
for action in simulation:
if action == 'C':
if available_chairs > 0:
available_chairs -= 1
else:
total_chairs += 1
elif action == 'R':
available_chairs += 1
elif action == 'U':
if available_chairs > 0:
available_chairs -= 1
else:
total_chairs += 1
elif action == 'L':
available_chairs += 1
results.append(total_chairs)
return results
# Example usage:
simulations = ["CCRUCL", "CRUC", "CCCC"]
print(minChairs(simulations)) # Output: [3, 2, 4]
This function processes each simulation string and calculates the minimum number of chairs needed.
Problem Statement #2 – Accenture Coding Test
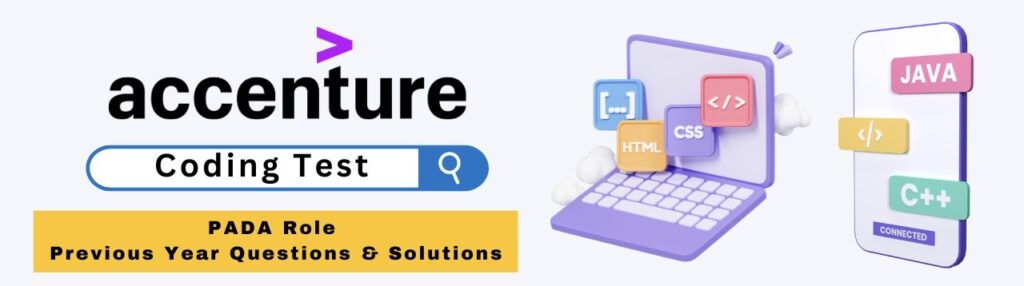
Function Description
Complete the function maxSubsetSum
in the editor below.
maxSubsetSum
has the following parameter(s):
int arr[]
: an array of integers
Returns:
long[]
: the sums calculated for eacharr[i]
Input Format – Accenture Coding Test
Input from stdin will be processed as follows and passed to the function:
- The first line contains an integer
n
, the number of elements inarr
. - Each of the next
n
lines contains an integer describingarr[i]
.
Constraints
- 1 ≤ n ≤ 100
- 1 ≤ arr[i] ≤ 10^4
Output Format
Return an array where each element is the sum of the factors of the corresponding element in the input array.
Sample Input
2
2
4
Sample Output
3
7
Explanation
- The factors of
arr[0] = 2
are[1, 2]
and their sum is3
. - The factors of
arr[1] = 4
are[1, 2, 4]
and their sum is7
.
Solution In Python 3 – Accenture Coding Test
def maxSubsetSum(arr):
def sum_of_factors(num):
factors_sum = 0
for i in range(1, num + 1):
if num % i == 0:
factors_sum += i
return factors_sum
results = [sum_of_factors(num) for num in arr]
return results
# Reading input
if __name__ == "__main__":
import sys
input = sys.stdin.read
data = input().split()
n = int(data[0])
arr = [int(data[i]) for i in range(1, n + 1)]
result = maxSubsetSum(arr)
for res in result:
print(res)
Explanation – Accenture Coding Test
- Function Definition:
maxSubsetSum(arr)
: This function takes an array of integers and returns an array of the sums of their factors.sum_of_factors(num)
: This helper function calculates the sum of all factors of a given numbernum
.
- Helper Function (
sum_of_factors
):- Iterates from
1
tonum
(inclusive). - Adds
i
tofactors_sum
ifi
is a factor ofnum
.
- Iterates from
- Main Logic:
- Reads input values from stdin.
- Constructs the array
arr
from input. - Calls
maxSubsetSum
with the arrayarr
and prints each result.
Sample Usage
For the input:
2
2
4
The output will be:
3
7
Each number in the input array has its factors summed and the results are printed line by line.
Problem Statement #3 – Accenture Coding Test
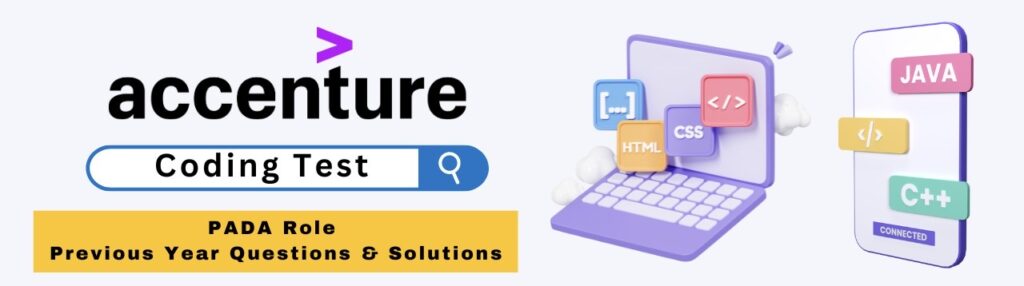
You need to compress a given string by describing the total number of consecutive occurrences of each character next to it. For example, given the string “abaasass”:
- ‘a’ occurs one time.
- ‘b’ occurs one time.
- ‘a’ occurs two times consecutively.
- ‘s’ occurs one time.
- ‘a’ occurs one time.
- ‘s’ occurs two times consecutively.
If a character only occurs once, it is added to the compressed string. If it occurs consecutive times, the character is added to the string followed by an integer representing the number of consecutive occurrences. Thus, the compressed form of the string “abaasass” is “aba2sas2”.
Function Signature – Accenture Coding Test
def compressedString(message: str) -> str:
pass
Input
message
(string): The string to be compressed.
Output
- Returns a string representing the compressed form of the input message.
Example
Input
abc
Output
abc
Input
abaasass
Output
aba2sas2
Solution In Python 3 – Accenture Coding Test
To solve this problem, we’ll iterate through the given string and keep track of consecutive characters and their counts. When the character changes, we’ll append the character and its count (if greater than 1) to the result string.
Here’s a step-by-step explanation of the implementation:
- Initialize an empty list
result
to build the compressed string. - Keep track of the current character and its count.
- Iterate through the string starting from the second character.
- If the current character is the same as the previous one, increment the count.
- If the current character is different, append the previous character and its count (if greater than 1) to the result list, then reset the count.
- After the loop, append the last character and its count (if greater than 1) to the result list.
- Join the result list into a string and return it.
Here is the complete implementation:
def compressedString(message):
if not message:
return ""
result = []
current_char = message[0]
count = 1
for char in message[1:]:
if char == current_char:
count += 1
else:
result.append(current_char)
if count > 1:
result.append(str(count))
current_char = char
count = 1
# Append the last character and its count
result.append(current_char)
if count > 1:
result.append(str(count))
return ''.join(result)
# Example usage
if __name__ == "__main__":
message = input().strip() # Read input
compressed_message = compressedString(message)
print(compressed_message)
Explanation – Accenture Coding Test
Let’s walk through the example message = "abaasass"
:
- Initialization:
result = []
current_char = 'a'
count = 1
- Iteration:
char = 'b'
: Append'a'
toresult
, updatecurrent_char = 'b'
,count = 1
char = 'a'
: Append'b'
toresult
, updatecurrent_char = 'a'
,count = 1
char = 'a'
: Incrementcount = 2
char = 's'
: Append'a2'
toresult
, updatecurrent_char = 's'
,count = 1
char = 'a'
: Append's'
toresult
, updatecurrent_char = 'a'
,count = 1
char = 's'
: Append'a'
toresult
, updatecurrent_char = 's'
,count = 1
char = 's'
: Incrementcount = 2
- Final Append:
- Append
's2'
toresult
- Join and Return:
result = ['a', 'b', 'a', '2', 's', 'a', 's', '2']
''.join(result)
results in'aba2sas2'
This produces the correct compressed string for the input “abaasass”.
Problem Statement – Accenture Coding Test
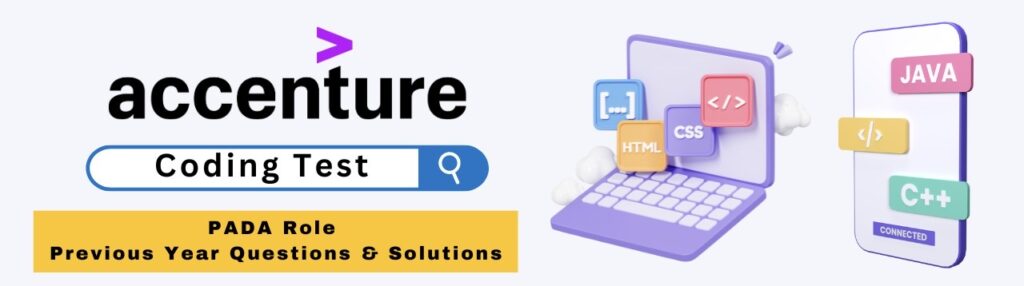
FC Codelona is trying to assemble a team from a roster of available players. They have a minimum number of players they want to sign, and each player needs to have a skill rating within a certain range. Given a list of players’ skill levels with desired upper and lower bounds, determine how many teams can be created from the list.
Function Signature:
def countTeams(skills: List[int], minPlayers: int, minLevel: int, maxLevel: int) -> int:
Parameters:
skills (List[int])
: An array of integers representing the skill levels of players.minPlayers (int)
: The minimum number of team members required.minLevel (int)
: The lower limit for skill levels, inclusive.maxLevel (int)
: The upper limit for skill levels, inclusive.
Returns:
int
: The total number of teams that can be formed per the criteria.
Example:
skills = [12, 4, 6, 13, 5, 10]
minPlayers = 3
minLevel = 4
maxLevel = 10
print(countTeams(skills, minPlayers, minLevel, maxLevel)) # Output: 5
Explanation:
- The list includes players with skill levels
[12, 4, 6, 13, 5, 10]
. - They want to hire at least 3 players with skill levels between 4 and 10, inclusive.
- Four of the players with the following skill levels
{4, 6, 5, 10}
meet the criteria. - There are 5 ways to form a team of at least 3 players:
{4, 5, 6}
,{4, 6, 10}
,{4, 5, 10}
,{5, 6, 10}
, and{4, 5, 6, 10}
. - Return 5.
Solution In Python 3 – Accenture Coding Test
To solve this problem, we need to:
- Filter the list of players to only include those whose skill levels are within the specified range.
- Count the number of ways to form teams with at least
minPlayers
members from the filtered list of players.
We can leverage the mathematical combinations (binomial coefficients) to efficiently count the number of ways to form teams of a given size. This approach avoids the need to explicitly generate all possible combinations.
Here’s the solution:
from math import comb
from typing import List
def countTeams(skills: List[int], minPlayers: int, minLevel: int, maxLevel: int) -> int:
# Filter the players based on the skill level range
valid_players = [skill for skill in skills if minLevel <= skill <= maxLevel]
total_teams = 0
n = len(valid_players)
# Calculate combinations for each team size from minPlayers to n
for team_size in range(minPlayers, n + 1):
total_teams += comb(n, team_size)
return total_teams
# Example Usage
skills = [12, 4, 6, 13, 5, 10]
minPlayers = 3
minLevel = 4
maxLevel = 10
print(countTeams(skills, minPlayers, minLevel, maxLevel)) # Output: 5
# Sample Input 0
skills = [4, 8, 5, 6]
minPlayers = 1
minLevel = 5
maxLevel = 7
print(countTeams(skills, minPlayers, minLevel, maxLevel)) # Output: 3
# Sample Input 1
skills = [4, 8, 5, 6]
minPlayers = 2
minLevel = 5
maxLevel = 7
print(countTeams(skills, minPlayers, minLevel, maxLevel)) # Output: 1
Explanation – Accenture Coding Test
- Filtering Valid Players:
- We filter the
skills
list to include only those players whose skill levels fall within the range[minLevel, maxLevel]
.
- Using Mathematical Combinations:
- For each possible team size from
minPlayers
to the total number of valid players, we calculate the number of ways to chooseteam_size
players from the valid players using thecomb
function from themath
module. - The
comb(n, k)
function computes the binomial coefficient, which is the number of ways to choosek
items fromn
items without regard to the order.
- Summing Up:
- We sum up the number of valid combinations for each possible team size to get the total number of teams that can be formed.
This approach is efficient as it leverages mathematical calculations to count combinations directly, avoiding the overhead of generating and iterating through all combinations.
Problem Statement #4 – Accenture Coding Test
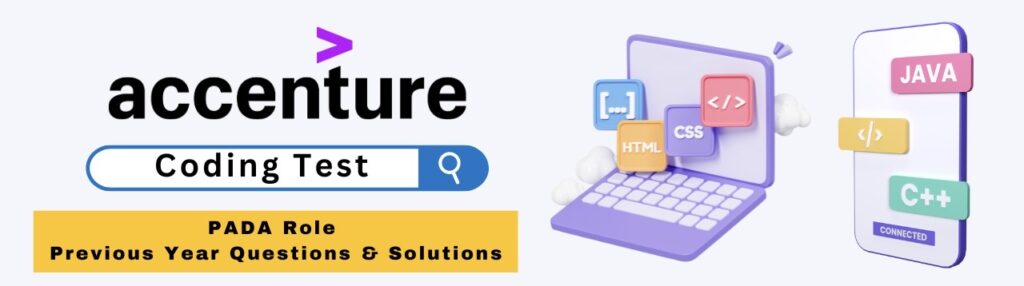
Given an array of integers, for each element determine whether that element occurs earlier in the array and whether it occurs later in the array. Create two strings of binary digits. In the first string, each character is a 1
if the value at that index also occurs earlier in the array, or 0
if not. In the second string, each character is a 1
if the value at that index occurs later in the array, or 0
if not. Return an array of two strings where the first string is related to lower indices and the second to higher indices.
Example
num = [1, 2, 3, 2, 1]
i | num[i] | num[0:i-1] | num[i+1:n-1] | string 1 | string 2 |
---|---|---|---|---|---|
0 | 1 | NULL | [2, 3, 2, 1] | 0 | 1 |
1 | 2 | [1] | [3, 2, 1] | 0 | 1 |
2 | 3 | [1, 2] | [2, 1] | 0 | 0 |
3 | 2 | [1, 2, 3] | [1] | 1 | 0 |
4 | 1 | [1, 2, 3, 2] | NULL | 1 | 0 |
For i = 0, the value 1 does not occur earlier in the array, but it does occur later. The two strings are now ['0', '1']
. For i = 1, the value 2 does not occur earlier in the array, but it does occur later. The two strings are now ['00', '11']
. For i = 2, the value 3 does not occur earlier or later in the array. The two strings are now ['000', '110']
. After similar analyses on the remaining values, the return array is ['00011', '11000']
.
Function Description
Complete the function bitPattern
as described below.
Parameters
int num[n]
: An array of integers.
Returns
string[2]
: An array of 2 strings as described.
Sample Input – Accenture Coding Test
num = [1, 3, 2, 3, 4, 1]
Sample Output
['000101', '110000']
Explanation
num = [1, 3, 2, 3, 4, 1]
i | num[i] | num[0:i-1] | num[i+1:n-1] | string 1 | string 2 |
---|---|---|---|---|---|
0 | 1 | NULL | [3, 2, 3, 4, 1] | 0 | 1 |
1 | 3 | [1] | [2, 3, 4, 1] | 0 | 1 |
2 | 2 | [1, 3] | [3, 4, 1] | 0 | 0 |
3 | 3 | [1, 3, 2] | [4, 1] | 1 | 0 |
4 | 4 | [1, 3, 2, 3] | [1] | 0 | 0 |
5 | 1 | [1, 3, 2, 3, 4] | NULL | 1 | 0 |
Solution – Accenture Coding Test
Here is the Python solution to the problem:
def bitPattern(num):
n = len(num)
earlier = ['0'] * n
later = ['0'] * n
for i in range(n):
for j in range(i):
if num[j] == num[i]:
earlier[i] = '1'
break
for j in range(i + 1, n):
if num[j] == num[i]:
later[i] = '1'
break
return [''.join(earlier), ''.join(later)]
# Sample Input
num = [1, 3, 2, 3, 4, 1]
print(bitPattern(num)) # Output: ['000101', '110000']
Explanation of the Solution – Accenture Coding Test
- Initialization: Accenture Coding Test
- We initialize two lists,
earlier
andlater
, both filled with ‘0’ initially, representing the binary strings.
- Iterating through the array:
- For each element in the array (
num[i]
):- We check if it has appeared earlier in the array (
num[0:i]
). If it has, we setearlier[i]
to ‘1’. - We also check if it appears later in the array (
num[i+1:n]
). If it does, we setlater[i]
to ‘1’.
- We check if it has appeared earlier in the array (
- Combining the results:
- We convert the
earlier
andlater
lists to strings and return them as the result.
This approach ensures that each element is checked against all previous and subsequent elements in the array to determine its presence, thus forming the required binary strings.
Sure, here’s a properly formatted version of the question, solution, and explanation:
Question #5 – Accenture Coding Test
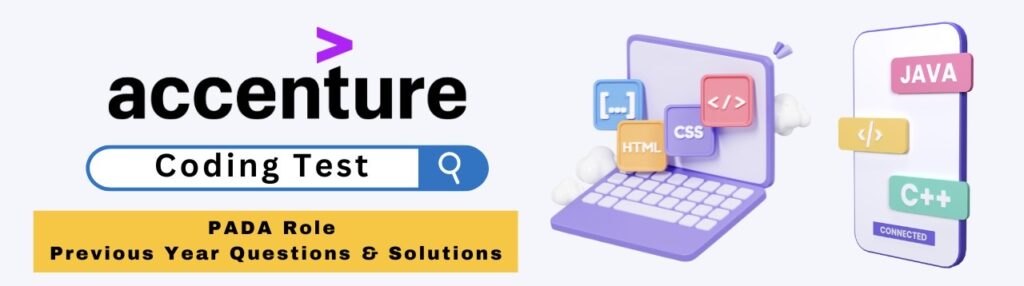
Consider a pair of integers, (a, b). The following operations can be performed on (a, b) in any order, zero or more times:
(a, b) -> (a + b, b)
(a, b) -> (a, a + b)
Return a string that denotes whether or not (a, b) can be converted to (c, d) by performing the operations zero or more times.
Function Signature: Accenture Coding Test
def isPossible(a: int, b: int, c: int, d: int) -> str:
Parameters:
a
(int): The first value in the pair (a, b).b
(int): The second value in the pair (a, b).c
(int): The first value in the pair (c, d).d
(int): The second value in the pair (c, d).
Returns:
str
: Return “Yes” if (a, b) can be converted to (c, d) by performing zero or more of the operations specified above, or “No” if not.
Examples – Accenture Coding Test
Example 1
Input:
a = 1
b = 4
c = 5
d = 9
Output:
"Yes"
Explanation: Accenture Coding Test
Convert (1, 4) to (5, 9) by performing the following sequence of operations:
(1, 4) -> (5, 4)
(5, 4) -> (5, 9)
Example 2
Input:
a = 1
b = 2
c = 3
d = 6
Output:
"No"
Explanation:
Attempt to convert (1, 2) to (3, 6). The possible paths toward the goal do not include the target (3, 6). Therefore, the answer is “No”.
Solution In Python 3 – Accenture Coding Test
def isPossible(a, b, c, d):
while c > a and d > b:
if c > d:
c -= d
else:
d -= c
if c == a and d == b:
return "Yes"
if c == a and d > b and (d - b) % a == 0:
return "Yes"
if d == b and c > a and (c - a) % b == 0:
return "Yes"
return "No"
# Test cases
print(isPossible(1, 4, 5, 9)) # Output: Yes
print(isPossible(1, 2, 3, 6)) # Output: No
Explanation – Accenture Coding Test
- Backward Operations: We apply the reverse operations to see if we can reach (a, b) from (c, d).
- If
c > d
, we perform(c, d) -> (c - d, d)
. - If
d > c
, we perform(c, d) -> (c, d - c)
.
- If
- Termination Conditions:
- If we exactly reach
(a, b)
, we return “Yes”. - If we get
c == a
andd > b
, we check if(d - b) % a == 0
to ensure thatd
can be reached by addinga
multiple times. - If we get
d == b
andc > a
, we check if(c - a) % b == 0
to ensure thatc
can be reached by addingb
multiple times. - If none of these conditions are met, we return “No”.
- If we exactly reach
This approach efficiently checks the reachability using mathematical operations, tracing the path back from (c, d) to (a, b) using modular arithmetic.