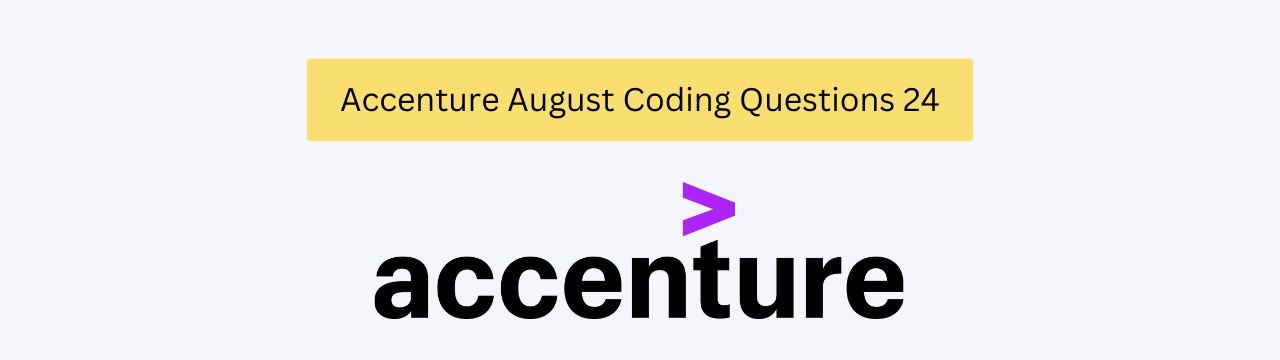
Accenture Coding Problems & Solutions | August 2024 Exam
In this post, we are discussing Accenture Coding Problems & its solutions from August 2024 tests.
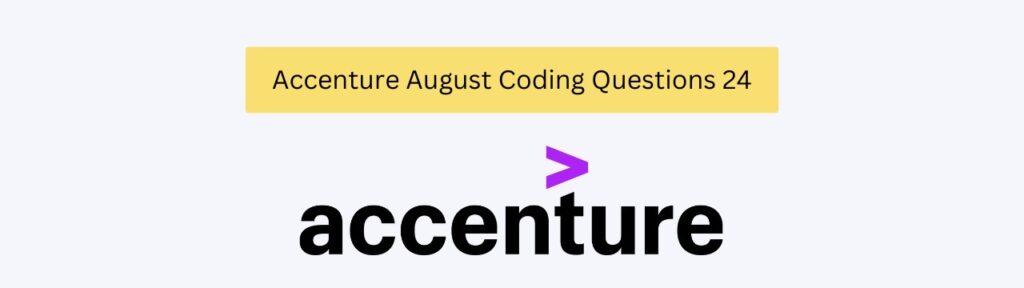
Accenture Coding Problems & Solutions
Problem: Find the Largest Element in an Array
Description:
You are given an array of integers. Your task is to find and return the largest element in the array.
Input Specification:
- An array of integers
arr
. - An integer
n
representing the number of elements in the array.
Output Specification:
- Return the largest integer from the array.
Example:
- Input:
arr = [1, 23, 5, 98, 45, 32]
,n = 6
- Output:
98
Solution in C
#include <stdio.h>
int findLargest(int arr[], int n) {
int max = arr[0];
for(int i = 1; i < n; i++) {
if(arr[i] > max) {
max = arr[i];
}
}
return max;
}
int main() {
int arr[] = {1, 23, 5, 98, 45, 32};
int n = sizeof(arr) / sizeof(arr[0]);
int largest = findLargest(arr, n);
printf("The largest element is: %d\n", largest);
return 0;
}
Explanation:
- We start by assuming the first element is the largest (
max = arr[0]
). - We then iterate through the array, and if we find an element greater than
max
, we updatemax
. - Finally,
max
is returned as the largest element.
Solution in Java
public class Main {
public static int findLargest(int[] arr, int n) {
int max = arr[0];
for(int i = 1; i < n; i++) {
if(arr[i] > max) {
max = arr[i];
}
}
return max;
}
public static void main(String[] args) {
int[] arr = {1, 23, 5, 98, 45, 32};
int n = arr.length;
int largest = findLargest(arr, n);
System.out.println("The largest element is: " + largest);
}
}
Explanation:
- Similar to the C solution, we assume the first element is the largest.
- We then iterate through the array and update
max
whenever we find a larger element. - The largest value is printed out at the end.
Solution in Python
def find_largest(arr, n):
max_val = arr[0]
for i in range(1, n):
if arr[i] > max_val:
max_val = arr[i]
return max_val
arr = [1, 23, 5, 98, 45, 32]
n = len(arr)
largest = find_largest(arr, n)
print("The largest element is:", largest)
Explanation: Accenture Coding Problems & Solutions
- We start by assuming the first element in the array is the largest.
- We iterate through the array, checking each element to see if it is larger than the current
max_val
. - If a larger element is found, we update
max_val
. - Finally, the largest element is returned.
Key Points: Accenture Coding Problems & Solutions
- Initialization: All solutions start by assuming the first element of the array is the largest.
- Iteration: Each element in the array is compared to the current largest element, and if a larger one is found, it becomes the new largest.
- Return: After scanning all elements, the largest value is returned.
This approach ensures that we efficiently find the largest element with a time complexity of O(n)
, where n
is the number of elements in the array.
Certainly! Below are examples of a simple problem, along with solutions provided in C, Java, and Python. Each solution is followed by an explanation.
Problem 2 : Find the Maximum Version
You are given an array of strings, where each string represents a filename with a version number. The filenames follow the format File_X
, where X
is a number representing the version. Your task is to find the highest version number among these filenames. If no filenames match the correct format, return -1
.
Example: Accenture Coding Problems & Solutions
- Input:
["File_1", "File_3", "File_2", "Invalid_File", "File_10"]
- Output:
10
Solution in C
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int findMaxVersion(char *files[], int size) {
int maxVersion = -1;
for (int i = 0; i < size; i++) {
if (strncmp(files[i], "File_", 5) == 0) {
char *versionStr = files[i] + 5;
int version = atoi(versionStr);
if (version > maxVersion) {
maxVersion = version;
}
}
}
return maxVersion;
}
int main() {
char *files[] = {"File_1", "File_3", "File_2", "Invalid_File", "File_10"};
int size = sizeof(files) / sizeof(files[0]);
int result = findMaxVersion(files, size);
printf("The highest version is: %d\n", result);
return 0;
}
Explanation: Accenture Coding Problems & Solutions
- strncmp: Compares the first 5 characters of the string to see if they match
"File_"
. - atoi: Converts the remaining part of the string to an integer.
- Loop: Iterates through the array, checking each string for the correct format and updating the maximum version if a higher one is found.
Solution in Java
public class Main {
public static int findMaxVersion(String[] files) {
int maxVersion = -1;
for (String file : files) {
if (file.startsWith("File_")) {
try {
int version = Integer.parseInt(file.substring(5));
if (version > maxVersion) {
maxVersion = version;
}
} catch (NumberFormatException e) {
// Ignore malformed versions
}
}
}
return maxVersion;
}
public static void main(String[] args) {
String[] files = {"File_1", "File_3", "File_2", "Invalid_File", "File_10"};
int result = findMaxVersion(files);
System.out.println("The highest version is: " + result);
}
}
Explanation: Accenture Coding Problems & Solutions
- startsWith: Checks if the string begins with
"File_"
. - substring: Extracts the part of the string after
"File_"
. - parseInt: Converts this substring to an integer.
- try-catch: Handles any potential
NumberFormatException
in case the string does not convert correctly.
Solution in Python
import re
def find_max_version(files):
max_version = -1
pattern = re.compile(r'^File_(\d+)$')
for file in files:
match = pattern.match(file)
if match:
version = int(match.group(1))
if version > max_version:
max_version = version
return max_version
if __name__ == "__main__":
files = ["File_1", "File_3", "File_2", "Invalid_File", "File_10"]
result = find_max_version(files)
print("The highest version is:", result)
Explanation: Accenture Coding Problems & Solutions
- re.compile: Compiles a regular expression pattern that matches the format
"File_X"
. - match: Applies this pattern to each string in the array.
- int: Converts the captured group (the version number) into an integer.
- Loop: Iterates through the list, finding and storing the highest version number found.
Summary: Accenture Coding Problems & Solutions
- C: We use string functions to manually check and extract the version number.
- Java: We leverage string manipulation and exception handling to find and parse the version number.
- Python: We use regular expressions for a concise solution to identify and extract the version number.
In all implementations, the logic checks if the filename starts with File_
and then extracts the version number, comparing it to keep track of the maximum version found. If no valid filenames are found, -1
is returned.